Set abModalVariant
abModalVariant
The Mulberry SDK Modal component can render itself in a variety of ways. To specify which format you’d like the modal to use by setting the abModalVariant property on the window object. You can do this like so:
window.abModalVariant = 11;
...
// the above must be set before initializing the modal
await mulberry.modal.init(...)
11
is the current standard modal version for showing Mulberry Unlimited subscription offers alongside regular plan offers for the best conversion. By setting this version before the modal is initialized, the modal will render for this specific design.
Suppressing Offers When Appropriate
To provide a quality UX to your customer, you’ll need to suppress Mulberry CTA’s and offers if the customer has already opted in to a subscription plan. To do this, the front end needs to understand if there is already a subscription in the cart. This can be accomplished in different ways, but is typically done by writing a function within your code base that is able to search the cart contents for the existence of a subscription plan.
Detecting if a user already has a subscription in the cart
const hasSubscription = async () => {
// The way you actually fetch the cart will depend on your platform
// and APIs. For simplicity here, we're assuming its a GET call to the /cart endpoint
const cart = await fetch('/cart');
return cart.items.some(item => item.metadata.warranty.plan_type === 'subscription')
}
Since the Mulberry SDK doesn't actually know about or have access to the cart contents, it needs to be told when there is a subscription in the cart. You can accomplish this by calling your hasSubscription()
function in combination with the mulberry.core.setHasSubscriptionInCart()
function as shown below:
mulberry.core.setHasSubscriptionInCart(await hasSubscription());
You'll want to make sure that you call the above function before you render Mulberry Inline or Modal components, as this will affect the way they render or whether or not they display themselves.
Inline Offer When Subscription is in cart
After you've told the Mulberry SDK that there is a subscription in the cart, Mulberry's Inline SDK component (if present on the page) will render as shown below.
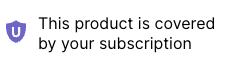
Modal Offer When Subscription is in cart
If you're rendering Mulberry's Modal SDK component and have told the SDK that there is a subscription in the cart, the SDK will automatically invoke the Modal's onWarrantyDecline()
callback so as to suppress the the modal from showing.