Overview
In-line offers are displayed directly on your eCommerce store in line with the contents of your PDP, cart or checkout page. In-line offers allow customers to select a warranty offer on the same real estate as the rest of your product and warranties will be added when the product is added to the cart.
An example of how to use the in-line offer is shown below:
Parameter | Definition | Required | Type |
---|---|---|---|
offers | Offers to display | yes | array |
settings | Retailer settings returned by the SDK | yes | object |
selector | An HTML class or ID to where the inline offer is to be appended. | yes | string |
placement | label for classifying the usage. Possible values are 'pdp' or 'cart' | no | string |
onWarrantyToggle | Callback that gets fired when a user toggles the warranty selection | yes | function |
layout | Configurations to alter the visual representation of the in-line | no | object |
Styling and Configuration
The in-line offer typically consists of the available protection plans, as well as the following elements:
ctaText
- a text headerfaq
an FAQ linkinput
type of user inputlogo
- the Mulberry branded logo
Each of these components can be toggled using the layout
section, where you can hide or show the different elements to suit your brand, as well as whether the offer buttons are vertically stacked or not. The following code snip creates an in-line offer that shows all of these elements and also visualizes the offer buttons in a vertical configuration:
/**
* Inline configuration options:
*
* ctaText: true|false (defaults to true)
* faq: true|false (defaults to true)
* input: 'buttons'|'dropdown'|'dropdown-standalone'|'radio' (defaults to 'buttons')
* logo: true|false (defaults to true)
* stacked: true|false (defaults to false)
*
*/
const inline = mulberry.inline.init({
layout: {
ctaText: true,
faq: true,
input: 'buttons',
logo: true,
stacked: true,
},
placement: 'pdp',
offers: warranties,
selector: '.mulberry-inline-container-alt1',
settings: settings,
onWarrantyToggle: (warranty) => {},
})
...
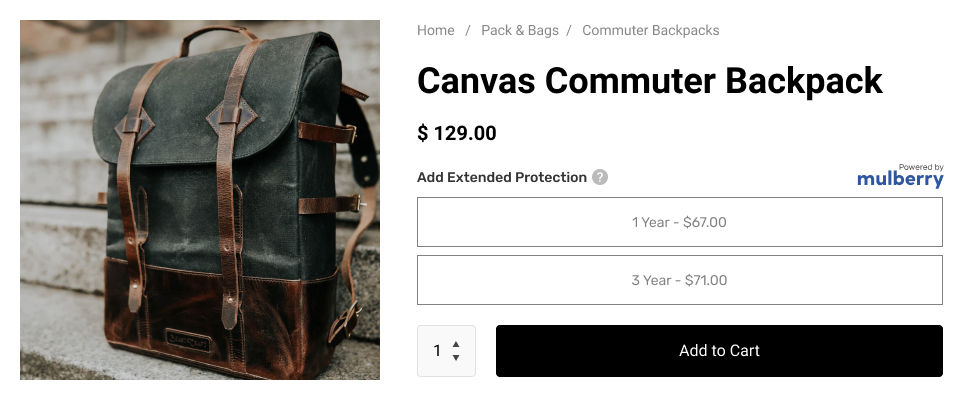
Alternatively, if we wanted to display the offer with only a text header of "Add Mulberry Product Protection", no logo or FAQ and horizontally aligned, we would use these settings:
const inline = mulberry.inline.init({
layout: {
faq: false,
logo: false,
},
Resulting in the following offer representation:
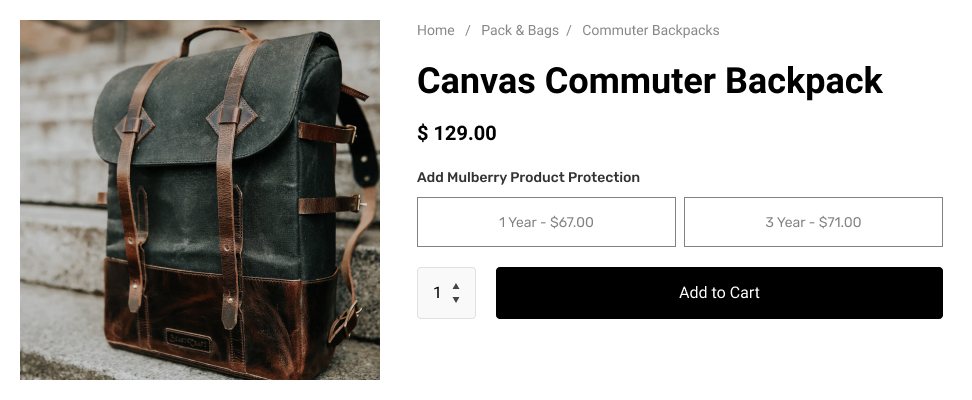
Please work with your Partner Success Manager to determine which elements work best for your brand to optimize conversion.
Input types
buttons
(default)
This appears as in the examples above, with each offer represented as a button.
dropdown
Options will appear as a dropdown with “Select a Plan” as the first option. When a user selects one of the plans, the same functionality is triggered as if an option button were pressed. If “Select a Plan” is re-selected, the previously selected option is de-selected.
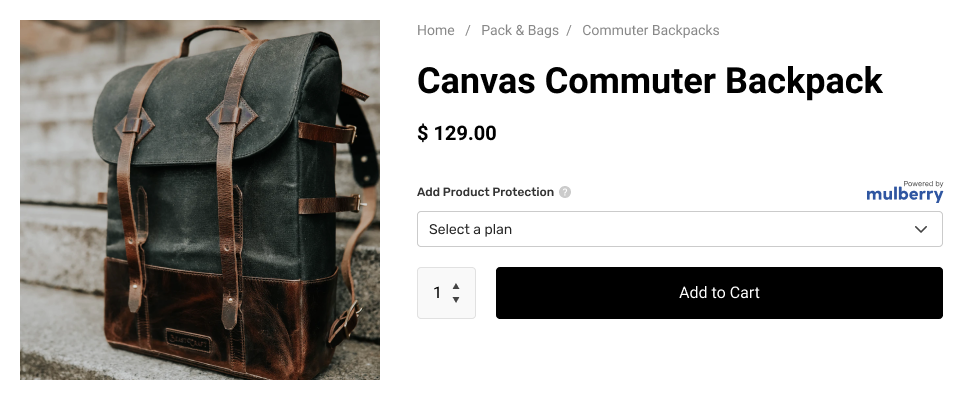
If this type is selected and only one offer is available, it will be displayed as a checkbox.
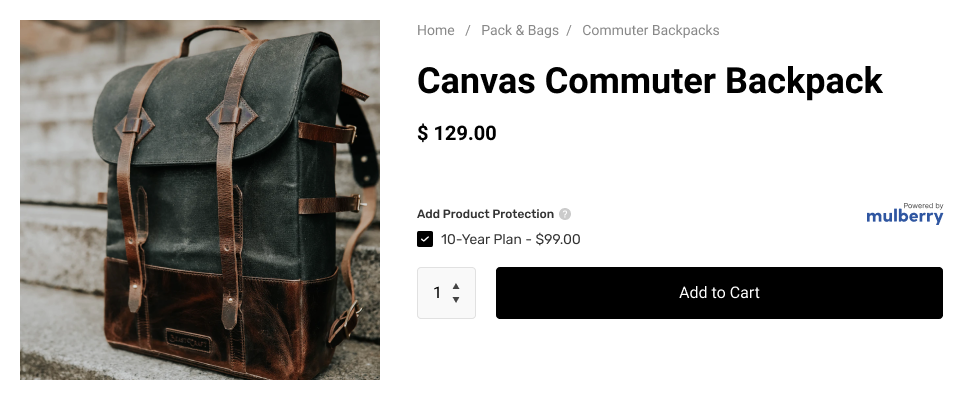
dropdown-standalone
Options will appear as a dropdown with the recommended plan pre-selected, and an “Add Plan” button to the right. No “Select a Plan” option is available. When an option is selected from the dropdown, no functional change is made other than displaying the selected option. When “Add Plan” is clicked, the selected plan is emitted with the onWarrantyToggle function and a success state is displayed. Plans cannot be un-added.
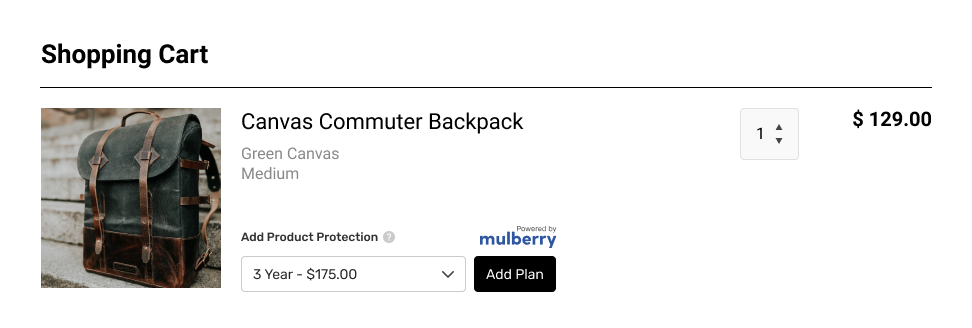
If this type is selected and only one offer is available, this will display as a button with plan details as text, such as “Add 5-Year Plan - $102.84.” If the single-plan button is clicked, it can be de-selected from the success state by clicking “Undo.”
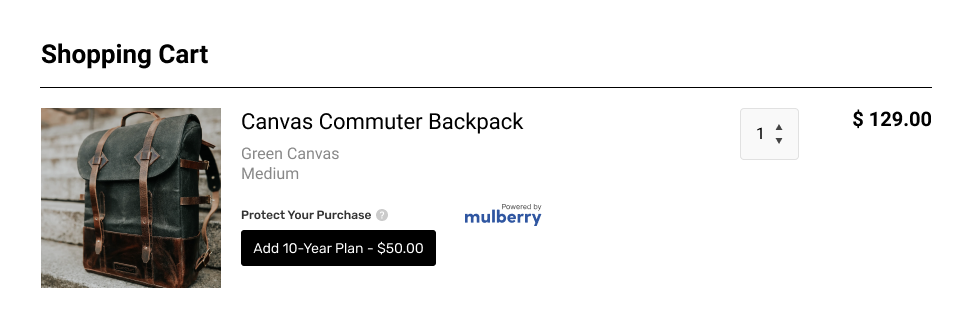
radio
Options will appear as stacked radio selections with an “Add Protection” button at the bottom. Functionally, this works the same as dropdown-standalone
regarding adding a plan, inability to deselect, and a single offer being displayed as a simple button.
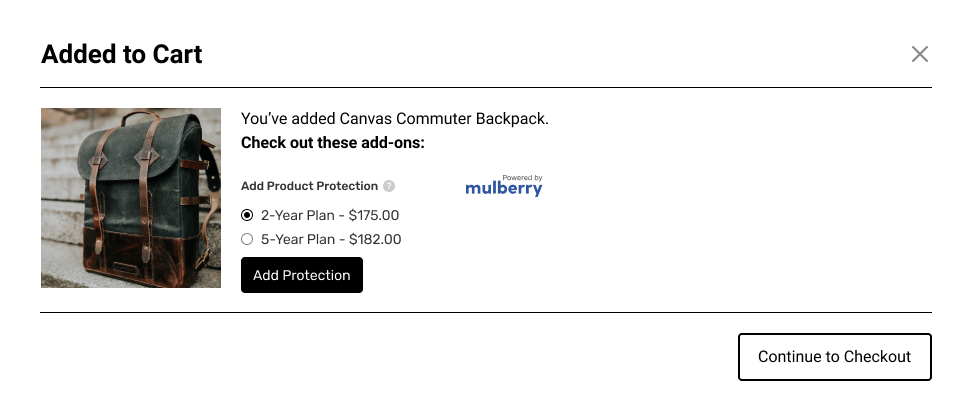
checkbox
Options will appear as stacked checkbox selections.
Resetting the inline
You can reset the the Inline to its original state at any time by calling deselectOffer()
on any Inline instance.
const inline = mulberry.inline.init(/*...*/);
// ...
// ...
// ...
inline.deselectOffer();